Introduction
In mobile game development, ensuring optimal performance is crucial for player experience. However, many developers overlook a critical aspect—device temperature. When a mobile device heats up due to prolonged usage or resource-heavy applications, the CPU throttles down to prevent overheating. As a result, this leads to a decline in game performance, often resulting in lower frame rates, stuttering, and slower response times.
In this blog post, we will explore how rising device temperatures impact CPU performance using Unity3D’s Profiler and a real-time temperature monitoring tool. We’ll compare the performance of a game in different temperature conditions, using screenshots from both the profiler and a temperature-monitoring app to visualize the impact of heat on game performance.
Additionally, for more insights on optimizing code performance, check out our previous blog post on Profiler.BeginSample
, which details how to use this method to analyze code execution time and identify bottlenecks in your scripts.
Code Sample for Performance Testing
We will use the following Unity C# script to simulate a heavy operation in the game. This will help us observe how temperature changes affect game performance. We will be using Profiler.BeginSample
to profile the execution time of the code.
using UnityEngine;
using UnityEngine.Profiling;
public class Example : MonoBehaviour
{
private void Update()
{
Profiler.BeginSample("Heavy Operation");
PerformHeavyOperation();
Profiler.EndSample();
}
private void PerformHeavyOperation()
{
for (int i = 0; i < 10000000; i++) { }
}
}
This simple script loops 10 million times, which allows us to measure how efficiently the CPU processes this heavy operation in different temperature conditions. As we progress, we’ll be analyzing the performance of this code on both a cold and hot device.
The Impact of Device Temperature on CPU Throttling
Mobile devices are designed to manage heat through thermal throttling. When the device’s temperature rises, the CPU’s clock speed is automatically reduced to prevent overheating. While this mechanism protects the hardware, it also results in slower processing and diminished performance in resource-intensive applications like games.
To better understand this, we will analyze two separate cases: one with a cold device and another with a hot device.
Case 1: Cold Device
Initial Device Temperature
We first monitor the initial temperature of the device before running the game using a mobile temperature-monitoring app. At this point, the following screenshot shows the device at an optimal operating temperature, which in this case is around 30°C.
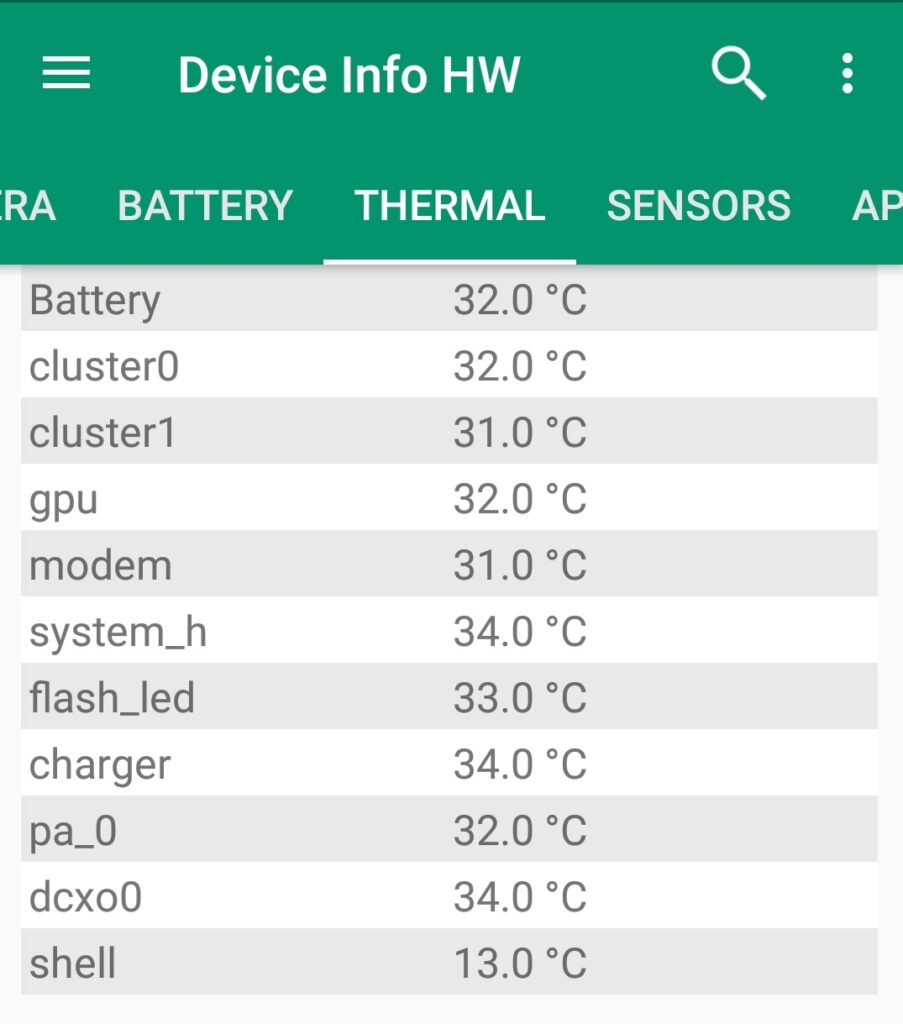
Performance Profiling on a Cool Device
Next, we use Unity’s Profiler to track how well the game performs when the device is cool. Using the code snippet from the previous section, we measure the performance of the heavy operation.
After running the game, we observe the performance metrics using Unity’s Profiler. As shown below, the screenshot displays the performance profiling results when the device is cool and running at its normal operating temperature, with no noticeable drops in performance.
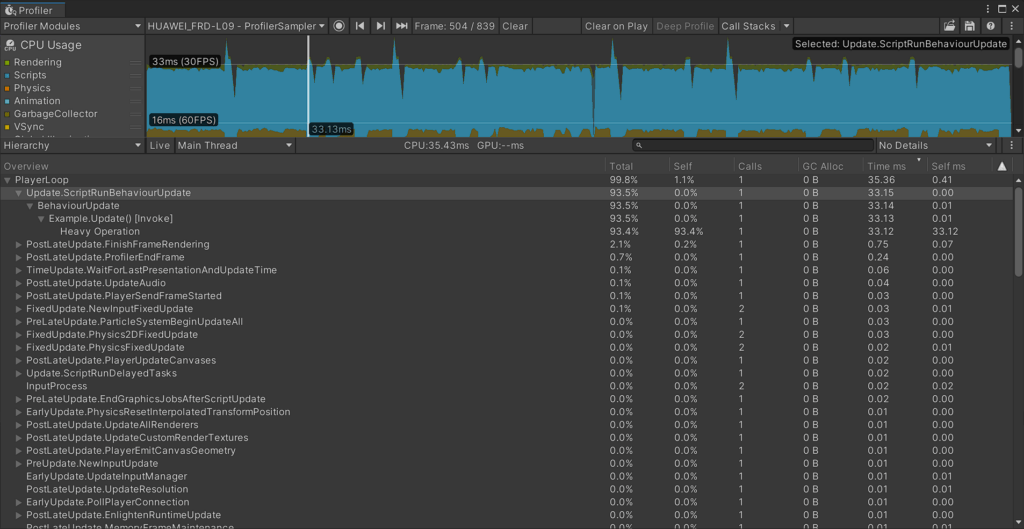
Case 2: Hot Device
Elevated Device Temperature
As the game continues running, the device heats up. Using the same temperature-monitoring application, we record the temperature, which has now increased to above 40°C. At this higher temperature, the CPU begins to throttle to manage the heat. The following screenshot shows the elevated temperature.
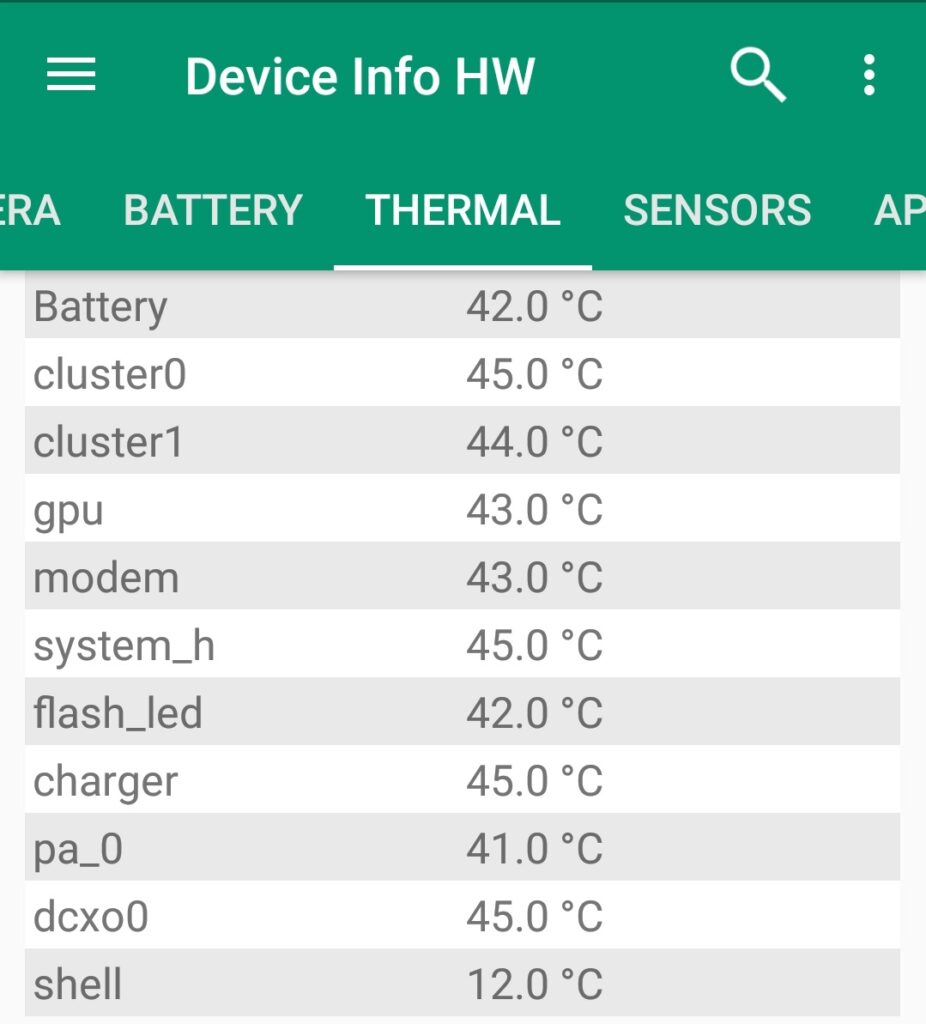
Performance Profiling on a Heated Device
With the device temperature elevated, we once again use Unity’s Profiler to examine the game’s performance. As a result, the profiling results now show a significant performance decline due to CPU throttling, with longer execution times and a drop in FPS.
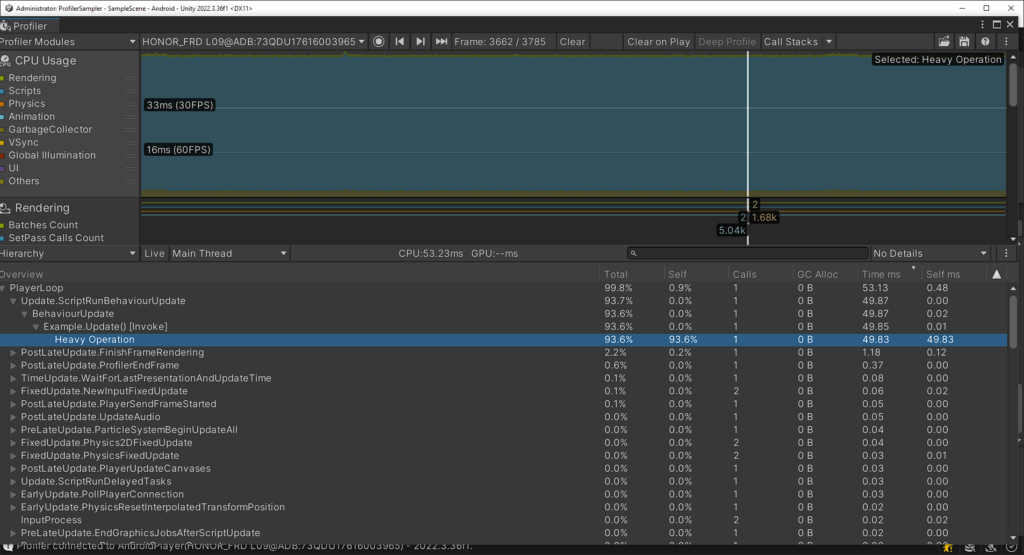
Conclusion
In conclusion, rising device temperatures directly affect game performance. When a device heats up, the CPU throttles down, resulting in slower code execution, lower FPS, and an overall degraded gaming experience.
To mitigate these issues, developers should consider the thermal characteristics of mobile devices. Techniques like reducing CPU usage, minimizing unnecessary calculations, and implementing adaptive performance strategies (where the game adjusts its resource demands based on device conditions) can help reduce the impact of thermal throttling.
By closely monitoring the device temperature and profiling key parts of the code, developers can ensure their games perform smoothly, even in demanding situations.
For further insights on optimizing your code for better performance, don’t forget to check our
blog post on Profiler.BeginSample
.