In my experience optimizing a Visual Novel game, I encountered a common issue: dynamically changing backgrounds consumed hundreds of megabytes of RAM. Why did this happen? The backgrounds were added to an array by reference, leading to excessive memory usage.
using System.Collections;
using UnityEngine;
public class BackgroundChanger : MonoBehaviour
{
[SerializeField] private SpriteRenderer spriteRenderer;
[SerializeField] private Sprite[] backgrounds;
private int currentIndex = 0;
void Start()
{
StartCoroutine(ChangeBackground());
}
IEnumerator ChangeBackground()
{
while (true)
{
spriteRenderer.sprite = backgrounds[currentIndex];
currentIndex = (currentIndex + 1) % backgrounds.Length;
yield return new WaitForSeconds(2f);
}
}
}
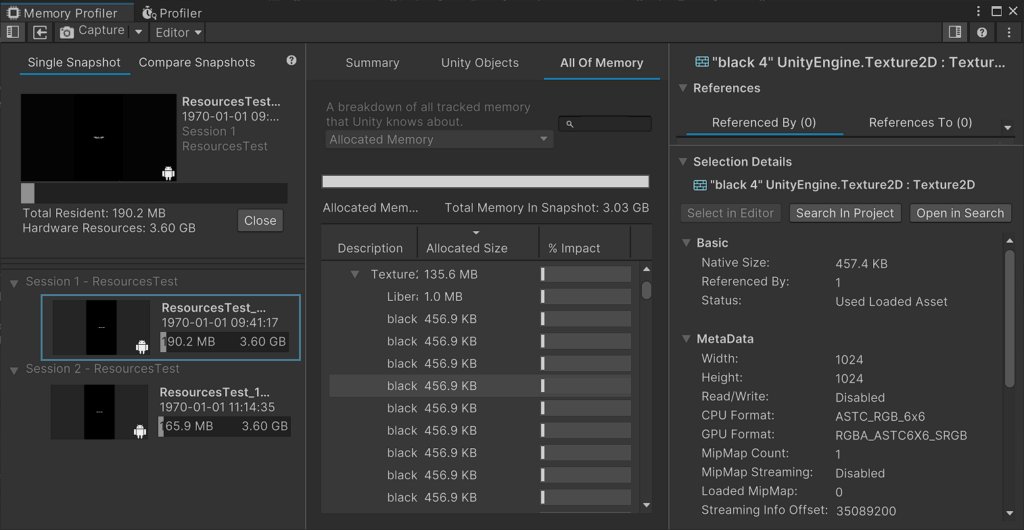
To resolve this problem, you only need to load the backgrounds that are currently needed. This can be accomplished using Resources.Load or AssetBundle.
In this article, I will focus on the first, simpler method.
using System.Collections;
using UnityEngine;
public class BackgroundChanger : MonoBehaviour
{
private const string SPRITE_NAME = "black";
[SerializeField]
private SpriteRenderer _spriteRenderer;
[SerializeField]
private float _waitTime = 2f;
private int _currentIndex = 1;
private Sprite _newSprite;
void Start()
{
StartCoroutine(ChangeBackground());
}
IEnumerator ChangeBackground()
{
while (true)
{
_newSprite = Resources.Load<Sprite>($"Graphics/{SPRITE_NAME} {_currentIndex}");
if (_newSprite != null)
{
_spriteRenderer.sprite = _newSprite;
_currentIndex++;
}
else
{
_currentIndex = 1;
}
yield return new WaitForSeconds(_waitTime);
Resources.UnloadUnusedAssets();
}
}
}
By implementing this solution, I significantly improved memory usage and reduced scene loading times. This change not only sped up the scene loading process but also saved a substantial amount of RAM. In my sample project, which contained dozens of graphics, these optimizations led to a noticeable boost in overall performance.
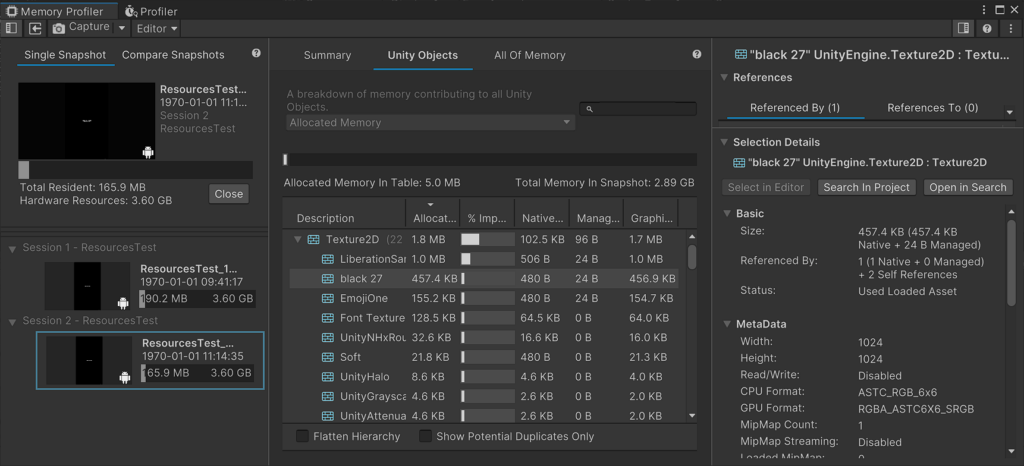
[…] back with our Visual Novel game. In the previous article, I discussed how textures can use a lot of RAM. This time, we’ll focus on sounds, specifically […]