Introduction
When working on a game in Unity, Debug.Log is a key debugging tool that helps track the code’s operation in real time. However, leaving Debug.Log calls in the final version of the game can significantly affect performance, especially on platforms like mobile devices and WebGL. In this post, I will discuss why excessive logging with Debug.Log happens and how to avoid performance issues.
How Debug Log Works
Debug.Log is used to display messages in the Unity console while the game is running. While this function is beneficial during development, if we leave these calls in production code, each log statement will consume system resources, particularly processor power.
Why Debug Log Lowers Performance
Every time Debug.Log is called, several key processes occur:
- Text Formatting – Unity has to process and format the text, especially when logging dynamic data like variables. This involves additional processor cycles, which can slow down your game.
- Blocking on the Main Thread – Debug.Log runs on the main thread, and excessive calls can block its execution. This results in significant drops in frames per second (FPS), ultimately compromising the smoothness of gameplay.
On devices like mobile and WebGL, excessive calls of this kind can significantly stress the processor and lower the frames per second (FPS).
Example: Debug Log in a Loop
Below is a screen from the profiler of a game running on the Android operating system, showing how much processor time is consumed by repeatedly calling Debug.Log
in a loop. As you can see, leaving these logs can lead to a significant drop in performance, especially on mobile platform.
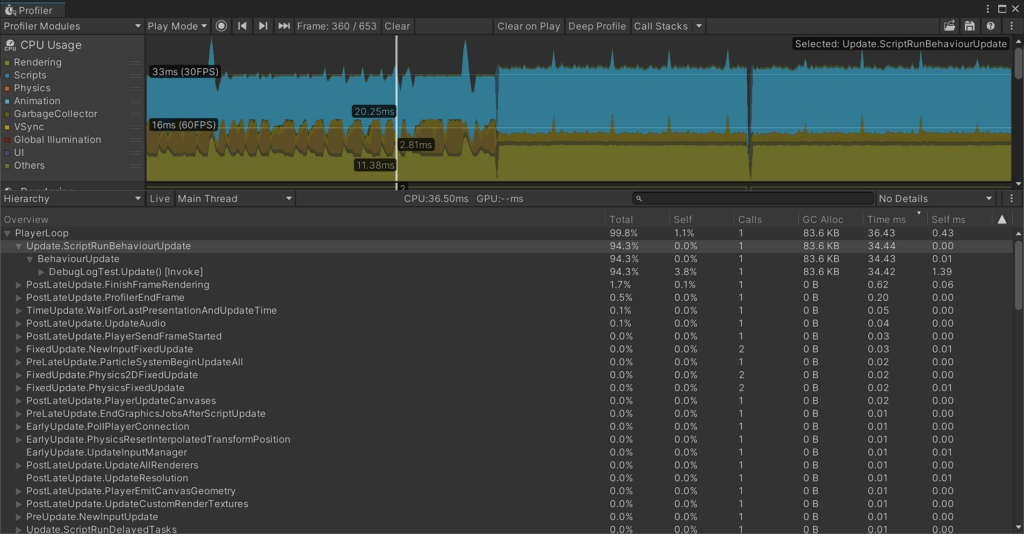
Here’s the code where Debug.Log
is called 100 times each frame:
using UnityEngine;
public class DebugLogTest : MonoBehaviour
{
private void Update()
{
for (int i = 0; i < 100; i++)
{
Debug.Log($"test");
}
}
}
How to Avoid This
To prevent performance issues caused by excessive logging, we can use the Conditional attribute along with a preprocessor directive to control whether Debug.Log is called only in debug versions, not in production builds.
We create a Logging
class with a Log
method and the attribute [System.Diagnostics.Conditional("ENABLE_LOG")]
, which means that the method will only be called if we define the preprocessor ENABLE_LOG
.
Here’s an example:
public static class Logging
{
[System.Diagnostics.Conditional("ENABLE_LOG")]
static public void Log(object message)
{
UnityEngine.Debug.Log(message);
}
}
With this solution, you can disable logging in production builds while keeping it in development or testing versions.
To use this method, call Logging.Log
instead of the standard Debug.Log
:
using UnityEngine;
public class DebugLogTest : MonoBehaviour
{
private void Update()
{
for (int i = 0; i < 100; i++)
{
Logging.Log($"test");
}
}
}
Adding a Custom Preprocessor Directive
To enable logging, you need to add the ENABLE_LOG
preprocessor in Unity’s project settings. You can do this by navigating to Player Settings -> Other Settings and adding ENABLE_LOG
in the Scripting Define Symbols field.
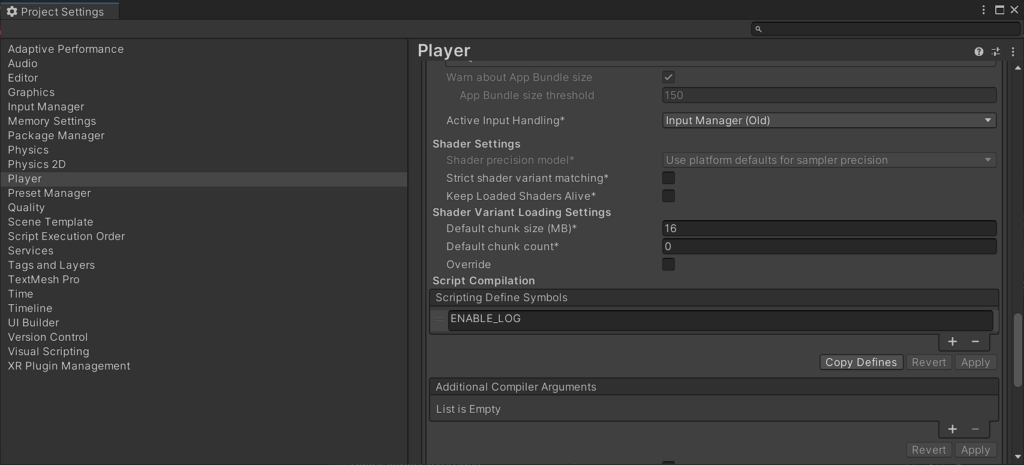
Conclusion
Debug.Log is a valuable tool during development, but leaving it in production code can lead to performance issues, particularly on mobile and WebGL platforms. Using the Conditional attribute and preprocessor directives allows you to control when logging is active, significantly improving the final game’s performance.
I hope this post helps you better manage logging in your Unity projects. If you have any questions or your own ways of optimizing code, feel free to share them in the comments!