Introduction
In game development, optimizing code is crucial for smooth gameplay. Particularly in Unity, where memory management and speed are of utmost importance, the method used to search and filter large data sets can significantly impact application performance. This article compares two methods of searching for elements in lists: using LINQ and traditional approaches. We will investigate how these techniques perform in scenarios that require fast filtering of large collections of data.
Sample Class: Character
Let’s start with the Character
class that represents a character object. Each character has an ID
and a CharacterType
(for example: Boss
or Npc
). This forms the basic structure of our data:
blic class Character
{
public int ID { private set; get; }
public CharacterType CharacterType { private set; get; }
public Character(int id, CharacterType characterType)
{
ID = id;
CharacterType = characterType;
}
}
public enum CharacterType
{
Boss,
Npc
}
Data Initialization
For testing purposes, we create a list of Character
objects with 100,000 randomly generated entries. Each object is assigned a random CharacterType
, giving us a realistic example of a large collection to filter.
private void InitData()
{
for (int i = 0; i < 100000; i++)
{
CharacterType characterType = (CharacterType)Random.Range(0, 1);
_characters.Add(new Character(i, characterType));
}
_matchingCharacters = new List<Character>(_characters.Count);
}
LINQ Without Optimization
Using LINQ without optimizations leads to significant memory allocations and increased garbage collection costs. Each call to .ToList()
creates a new list, which negatively impacts application performance, particularly when working with large datasets. This overhead can result in noticeable frame drops during gameplay, highlighting the importance of memory management in Unity.
private List<Character> FindCharacterByTypeLinqu(CharacterType characterType)
{
_matchingCharacters.Clear();
return _characters.Where(c => c.CharacterType == characterType).ToList();
}
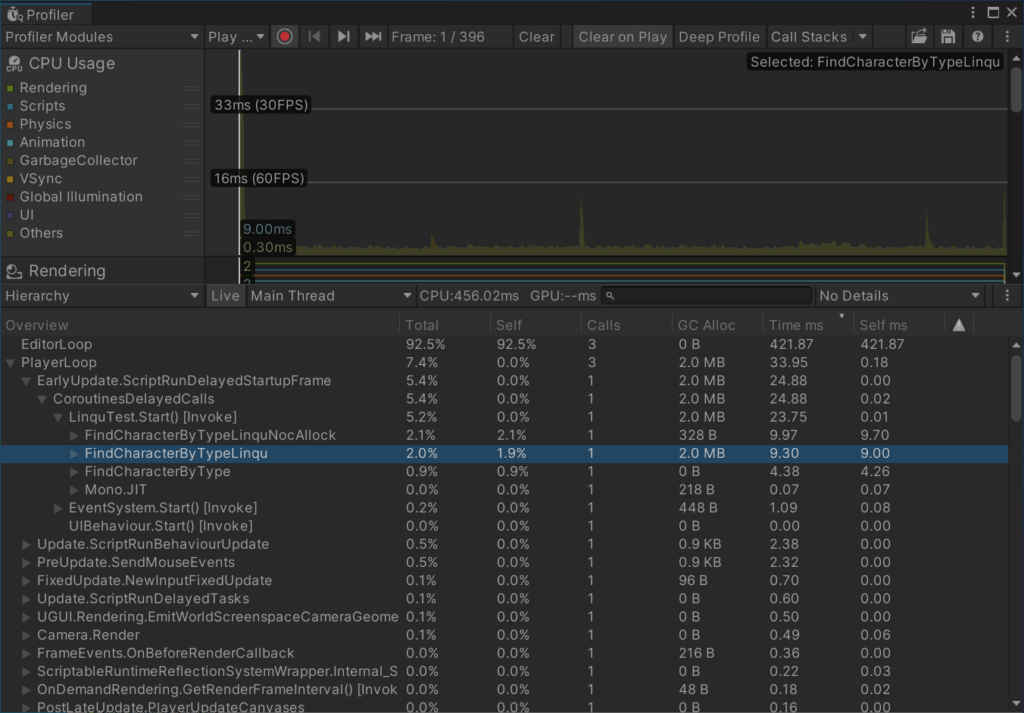
Optimized LINQ
By optimizing the LINQ method and removing the .ToList()
call, we see a noticeable improvement in memory efficiency. This adjustment allows us to manually add filtered elements to the _matchingCharacters
list, which significantly reduces memory overhead and the frequency of garbage collection calls. As a result, this optimized approach provides smoother performance, particularly beneficial when handling extensive collections of data.
private List<Character> FindCharacterByTypeLinquNocAllock(CharacterType characterType)
{
_matchingCharacters.Clear();
foreach (var character in _characters.Where(c => c.CharacterType == characterType))
{
_matchingCharacters.Add(character);
}
return _matchingCharacters;
}
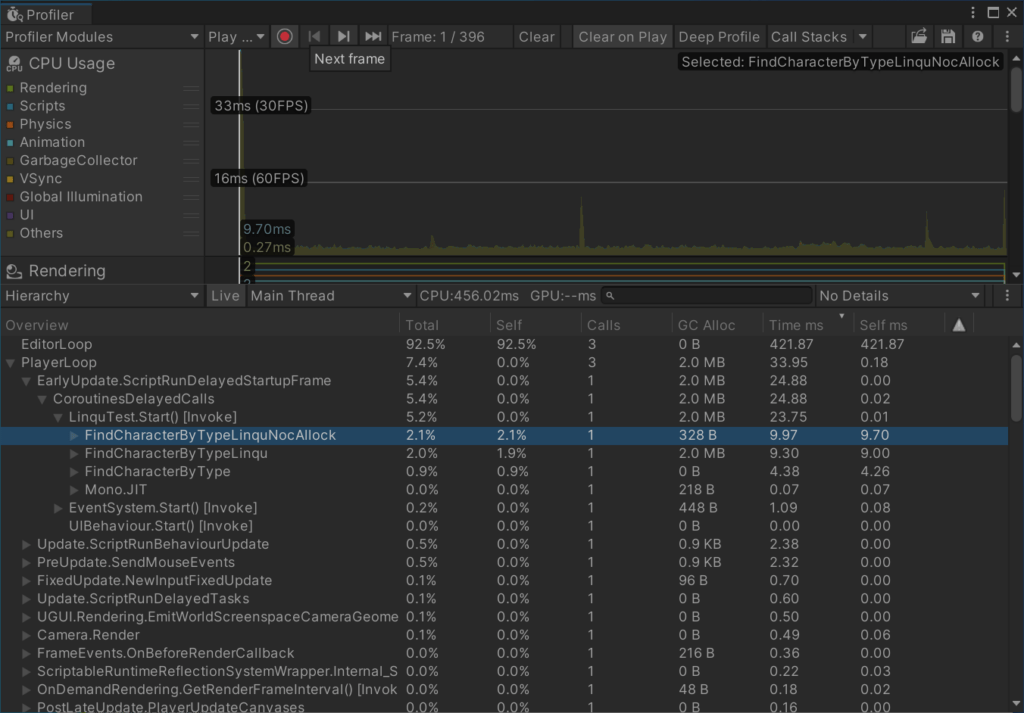
ToList()
in the LINQ method.Traditional List Searching
The traditional list searching method utilizes a simple for
loop, resulting in minimal memory allocations and the lowest memory management costs. This straightforward approach ensures that we check each character efficiently, allowing for maximum performance. Such efficiency is crucial in high-demand applications, where managing resources effectively can lead to smoother gameplay experiences.
private List<Character> FindCharacterByType(CharacterType characterType)
{
_matchingCharacters.Clear();
int charactersCount = _characters.Count;
Character currentCharacter = null;
for (int i = 0; i < charactersCount; i++)
{
currentCharacter = _characters[i];
if (currentCharacter.CharacterType == characterType)
{
_matchingCharacters.Add(currentCharacter);
}
}
return _matchingCharacters;
}
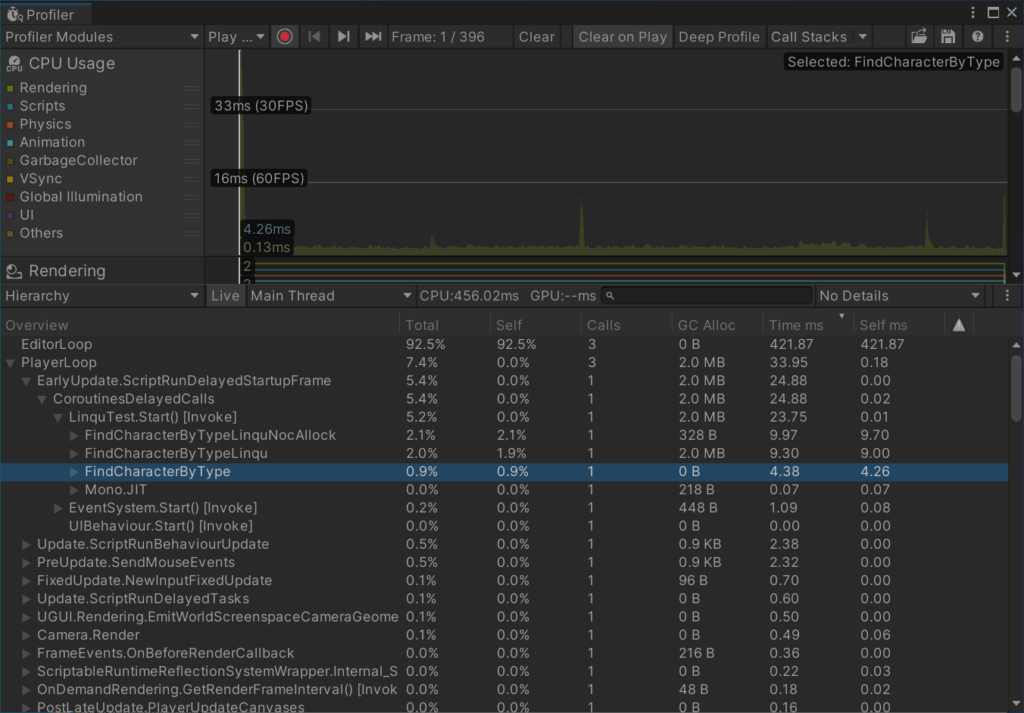
Conclusion
Test results clearly show that each approach has its advantages and disadvantages:
- LINQ without optimization is simple and readable but has a large impact on memory and performance, especially with large collections.
- Optimized LINQ still benefits from convenient syntax but becomes more efficient in terms of memory due to the removal of
.ToList()
. - Traditional list searching is by far the most resource-efficient approach, although less elegant than LINQ.
The choice of the optimal method depends on the project’s scale and the specific requirements of the task. In situations where performance is critical (e.g., on mobile devices), it is advisable to consider the traditional approach. Conversely, in projects where code readability is more important than a slight performance compromise, optimized LINQ can be a great choice.
By utilizing profiling and analysis, developers can select the method that best meets their project needs, ensuring smooth gameplay and enhancing the user experience.